Building a Currency Converter using OpenExchangeRates API and requests
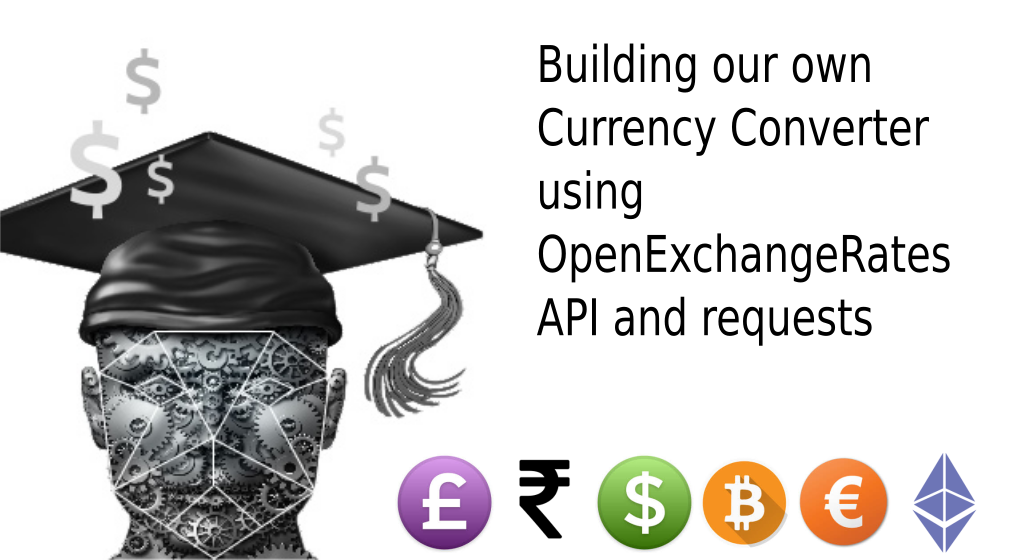
Converting currency in google is simple, no fun and no brainer. Let's leverage the use of Python and build a currency converter that will not only convert traditional currency but also digital currencies like BitCoin, Etherum and others.
We will be using OpenExchangeRates API for this. Sign Up and register for a new API Key.
To get a view of OpenExchangeRates API, replace YOUR_APP_ID with the key you get in a email in the following link and make a new request in browser. OpenExchanges
To make such request we will be using Requests. After getting a response we will fetch the rates and calculate necessary value as shown in the code below.
import requests
class CurrencyConverter:
def __init__(self, YOUR_APP_ID, symbols):
self.YOUR_APP_ID = YOUR_APP_ID
self.symbols = symbols
self._symbols = ",".join([str(s) for s in symbols])
r = requests.get(
"https://openexchangerates.org/api/latest.json",
params = {
"app_id" : self.YOUR_APP_ID,
"symbols" : self._symbols,
"show_alternatives": True
}
)
self.rates_ = r.json()["rates"]
self.rates_["USD"] = 1
def convert(self, value, symbol_from, symbol_to):
try:
return value * 1/self.rates_.get(symbol_from) * self.rates_.get(symbol_to)
except TypeError:
print("Error")
return None
if __name__ == "__main__":
YOUR_APP_ID = "3dccea5302c7430db25f6b8863300084"
# Enter all the rates you want in the list
cadUSDconverter = CurrencyConverter(YOUR_APP_ID, ["USD", "NPR"])
# Converts 10 US Dollars to Nepali Rupees.
print(cadUSDconverter.convert(10, 'USD', 'NPR'))
Converting BitCoin, Etherum and other Digital Currencies
If we made show_alternatives parameter True we can get rates for digital currencies like bitcoin, Etherum and others. Add all the necessary symbols required while creating new currency converter and we are all ready.
simpleConverter = CurrencyConverter(YOUR_APP_ID, ["USD", "NPR", "BTC"])
print(simpleConverter.convert(10, 'USD', 'BTC'))