How to create a dict in python?
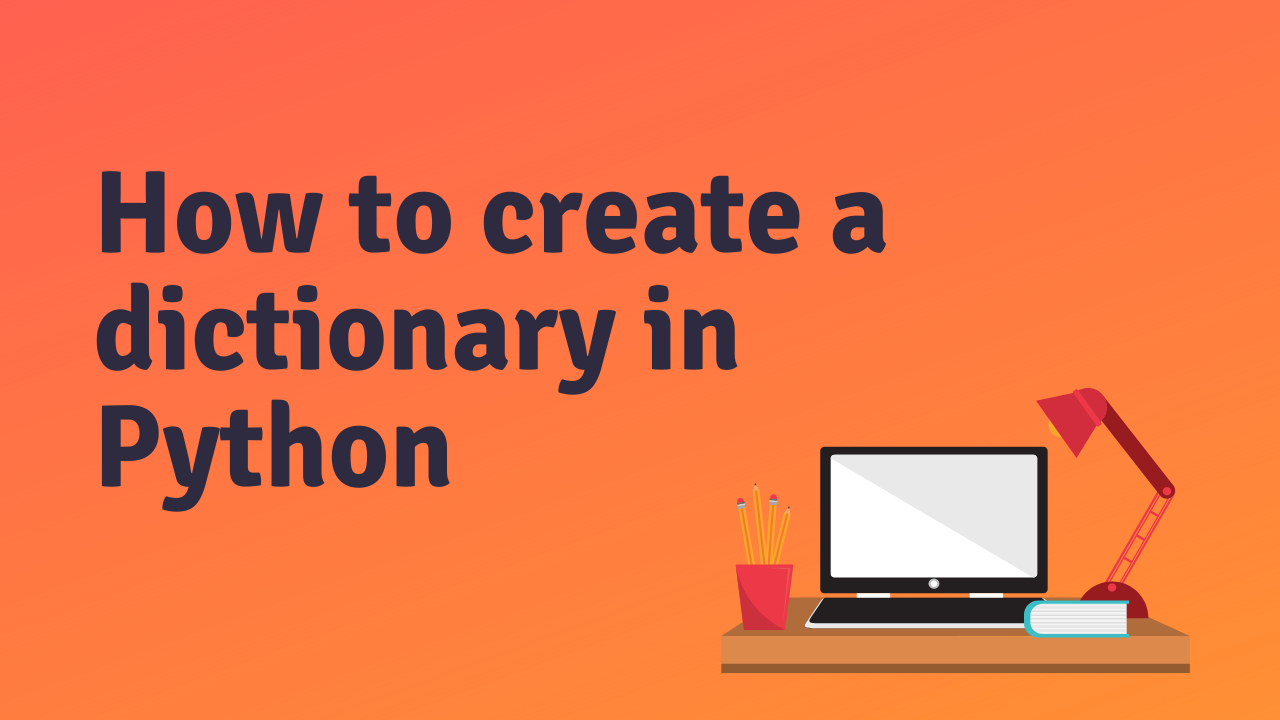
The dictionary is a fundamental data structure of a python ecosystem. It is written as a series of key:value pairs, separated by commas, enclosed in curly braces.
Example:
fruits = {"orange": 300, "apple": 500, "banana": 200}
How to create an empty dictionary in python?
To create an empty dictionary, we use the following syntax:
empty_dict = {}
another_empty_dict = dict()
How to create a dictionary with initial values?
To create a dictionary with initial values, we use the following syntax:
my_dict = {"key1": "value1", "key2": "value2"}
How to create a dictionary with initial values using the dict() function?
my_dict = dict("key1"="value1", "key2"="value2")
How to create a nested dictionary?
To create a nested dictionary, we use the following syntax:
my_dict = {"key1": {"key11": "value11", "key12": "value12"}, "key2": {"key21": "value21", "key22": "value22"}}
How to create a dictionary from a list?
To create a dictionary from a list, we use the following syntax:
my_dict = dict([("key1", "value1"), ("key2", "value2")])
How to create a dictionary from a list of tuples?
To create a dictionary from a list of tuples, we use the following syntax:
my_dict = dict([("key1", "value1"), ("key2", "value2")])
How to create a dictionary by passing a list of keys and a list of values?
To create a dictionary by passing a list of keys and a list of values, we use the following syntax:
my_dict = dict(keys=["key1", "key2"], values=["value1", "value2"])
How to create a dictionary by passing key/value tuple pairs to the type constructor?
To create a dictionary by passing key/value tuple pairs to the type constructor, we use the following syntax:
my_dict = dict(zip("abc", [1, 2, 3]))
How to create a dictionary from a dictionary?
To create a dictionary from a dictionary, we use the following syntax:
my_dict = dict({"key1": "value1", "key2": "value2"})
How to create an ordered dictionary?
To create an ordered dictionary, we use the following syntax:
from collections import OrderedDict
my_dict = OrderedDict({"key1": "value1", "key2": "value2"})