How to sort a dictionary in python: Sort a dictionary by key, value, key and value and reverse.
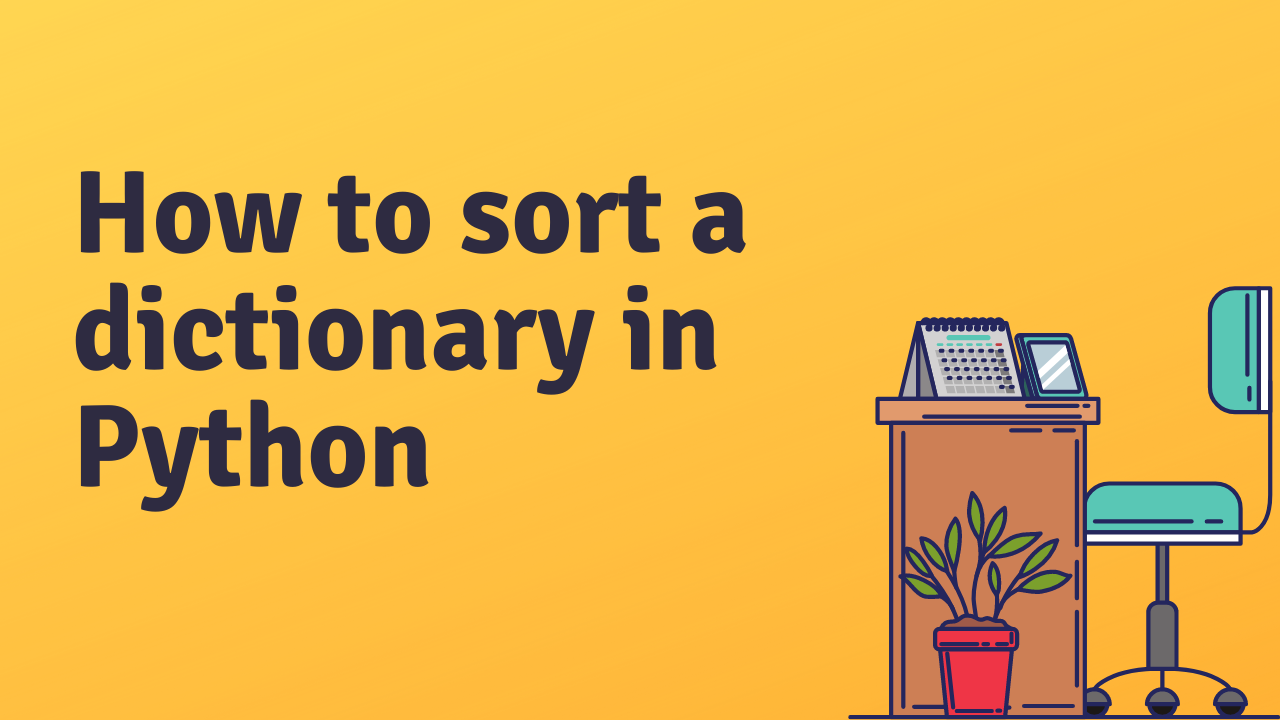
The dictionary is a fundamental data structure of a python ecosystem. It is written as a series of key:value pairs, separated by commas, enclosed in curly braces.
Example:
fruits = {"orange": 300, "apple": 500, "banana": 200}
If we sort fruits by key, we get:
{'apple': 500, 'banana': 200, 'orange': 300}
If we sort fruits by value, we get:
{'banana': 200, 'orange': 300, 'apple': 500}
Sorting a python dictionary
To get a sorted dictionary of key/value pairs, we need to do the following:
1. Convert the dictionary into a list of tuples (actually it's a view object not an actual list) by using the items() method.
fruits.items() will return a list of tuples as shown below:
dict_items([('orange', 300), ('apple', 500), ('banana', 200)])
2. Sort the list of tuples by using the sorted() function.
Now to sort the list of tuples we got from the items() method, we will use the sorted() function. Next, we need to define how to compare the tuples. We will use the key= argument to specify the key to sort by. The value of the key parameter should be a function that takes a single argument and returns a value to sort by. For example if we want to sort the list of tuples by the first element of the tuple, we can use the key=lambda x: x[0] argument. To sort the list by the the value i.e second element we will use:
sorted(fruits.items(), key=lambda x: x[1])
3. Convert the list of tuples back into a dictionary by using the dict() function.
To convert the sorted list of tuples back into a dictionary, we can use the dict() function.
dict(sorted(fruits.items(), key = lambda x: x[1]))
If we want to sort in reverse order, we can use the reverse=True argument.
Code Examples
def sort_dict(dictionary):
"""
Sort a dictionary by key.
"""
return dict(sorted(dictionary.items(), key=lambda x: x[0]))
def sort_dict_by_value(dictionary):
"""
Sort a dictionary by value.
"""
return dict(sorted(dictionary.items(), key=lambda x: x[1]))
def sort_dict_by_key_and_value(dictionary):
"""
Sort a dictionary by key and value.
"""
return dict(sorted(dictionary.items(), key=lambda x: (x[0], x[1])))
def sort_dict_by_value_and_key(dictionary):
"""
Sort a dictionary by value and key.
"""
return dict(sorted(dictionary.items(), key=lambda x: (x[1], x[0])))
def sort_dict_by_value_and_key_and_reverse(dictionary):
"""
Sort a dictionary by value and key and reverse.
"""
return dict(sorted(dictionary.items(), key=lambda x: (x[1], x[0]), reverse=True))
def sort_dict_by_key_and_value_and_reverse(dictionary):
"""
Sort a dictionary by key and value and reverse.
"""
return dict(sorted(dictionary.items(), key=lambda x: (x[0], x[1]), reverse=True))
# Examples
if __name__ == "__main__":
fruits = {"orange": 300, "olives": 400, "apple": 500, "banana": 200, "kiwi": 400}
print("Sort by key:")
print(sort_dict(fruits))
print("Sort by value:")
print(sort_dict_by_value(fruits))
print("Sort by key and value:")
print(sort_dict_by_key_and_value(fruits))
print("Sort by value and key:")
print(sort_dict_by_value_and_key(fruits))
print("Sort by value and key and reverse:")
print(sort_dict_by_value_and_key_and_reverse(fruits))
print("Sort by key and value and reverse:")
print(sort_dict_by_key_and_value_and_reverse(fruits))